Contents
Previous
Next
This >chapter >describes >many >of >the >widgets >that >
are >provided >with >FLTK > and >covers >how >to >query >
and >set >the >standard >attributes. >
FLTK >provides >many >types >of >buttons: >
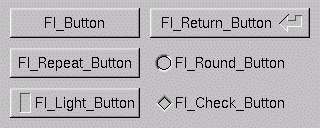
For >all >of >these >buttons >you >just >need >to >
include >the >corresponding > <FL/Fl_xyz_Button.H>>
header >file. > The >constructor >takes >the > bounding >
box >of >the >button >and >optionally >a >label >
string: >
>Fl_Button *button = new Fl_Button(x, y, width, height, "label");
>Fl_Light_Button *lbutton = new Fl_Light_Button(x, y, width, height);
>Fl_Round_Button *rbutton = new Fl_Round_Button(x, y, width, height, "label");
>
Each >button >has >an >associated >
type()> which >allows >it >to >behave >as >
a >push >button, >toggle > button, >or >radio >button: >
>button->type(0);
>lbutton->type(FL_TOGGLE_BUTTON);
>rbutton->type(FL_RADIO_BUTTON);
>
For >toggle >and >radio >buttons, >the >
value()> method >returns >the >current >button >
state >(0 >= >off, > 1 >= >on). > The >
set()> and > clear()>
methods >can >be >used >on >toggle >buttons >to >
turn >a > toggle >button >on >or >off, >respectively. >
Radio >buttons >can >be >turned >on > with >the >
setonly()> method; >this > will >also >turn >
off >other >radio >buttons >in >the >same >group. >
FLTK >provides >several >text >widgets >for >displaying >
and >receiving >text: >
The >Fl_Output> and >Fl_Multiline_Output>
widgets >allow > the >user >to >copy >text >from >the >
output >field >but >not >change >it. >
The >value()>
method > is >used >to >get >or >set >the >string >
that >is >displayed: >
>Fl_Input *input = new Fl_Input(x, y, width, height, "label");
>input->value("Now is the time for all good men...");
>
The >string >is >copied >to >the >widget's >own >
storage >when >you >set >the >value()> of >the >
widget. >
Unlike >text >widgets, >valuators >keep >track >of >
numbers >instead >of > strings. >FLTK >provides >the >
following >valuators: >
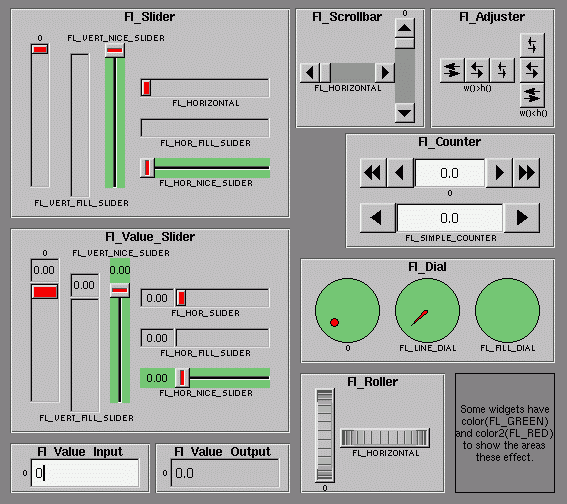
The >value()>
method >gets >and >sets >the >current >value >of >the >
widget. > The >
minimum()> and >
maximum()> methods >set >the >range >of >
values >that >are >reported >by > the >widget. >
The >Fl_Group> widget >class >is >used >as >a >
general >purpose > "container" >widget. > Besides >grouping >
radio >buttons, >the >groups >are > used >to >encapsulate >
windows, >tabs, >and >scrolled >windows. > The >following >
group >classes >are >available >with >FLTK: >
- Fl_Double_Window> - >
A >double-buffered >window >on >the >screen. >
- Fl_Gl_Window> - >An >
OpenGL >window >on >the >screen. >
- Fl_Group> - >The >base >
container >class; >can >be >used >to >group > any >
widgets >together. >
- Fl_Scroll> - >A >
scrolled >window >area. >
- Fl_Tabs> - >Displays >
child >widgets >as >tabs. >
- Fl_Window> - >A >window >
on >the >screen. >
The >size >and >position >of >widgets >is >usually >
set >when >you >create >them. >You >can >access >them >
with >the >x()>, >y()>, >w()>, >
and >h()> methods. >
You >can >change >the >size >and >position >by >
using >the >position()>, > resize()>, >
and >size()> methods: >
>button->position(x, y);
>group->resize(x, y, width, height);
>window->size(width, height);
>
If >you >change >a >widget's >size >or >position >
after >it >is >displayed >you >will >have >to >call >
redraw()> on >the >widget's >parent. >
FLTK >stores >the >colors >of >widgets >as >an >8-bit >
number >that >is >an >index >into >a >color >palette >
of >256 >colors. >This >is >not> the >X >or >
WIN32 >colormap, >but >instead >is >an >internal >table >
with >fixed >contents. >
There >are >symbols >for >naming >some >of >the >
more >common >colors: >
- FL_BLACK> (this >is >the >default >label >
color)>
- FL_RED>
- FL_GREEN>
- FL_YELLOW>
- FL_BLUE>
- FL_MAGENTA>
- FL_CYAN>
- FL_WHITE> (this >is >the >default >background >
color >of >text >widgets)>
- FL_GRAY> (this >is >the >default >background >
color >of >most >widgets)>
The >widget >color >can >be >set >using >the >
color()> method: >
>button->color(FL_RED);
>
Similarly, >the >label >color >can >be >set >using >
the >labelcolor()> method: >
>button->labelcolor(FL_WHITE);
>
The >type >Fl_Boxtype> stored >and >returned >
in > Fl_Widget::box()>
is >an >enumeration >defined >in >
<Enumerations.H>>: >
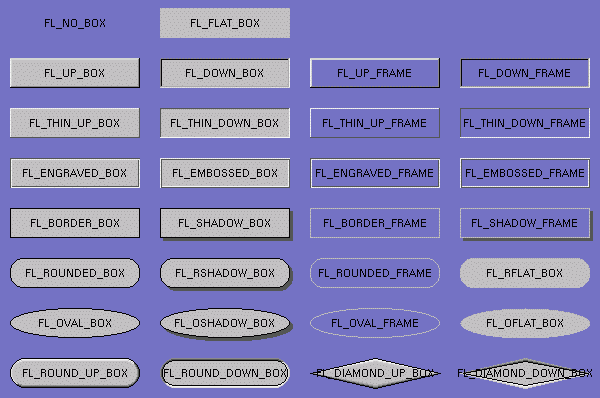
FL_NO_BOX> means >nothing >is >drawn >at >all, >
so >whatever >is > already >on >the >screen >remains. >
The >FL_..._FRAME> types >only > draw >their >
edges, >leaving >the >interior >unchanged. > In >the >
above >diagram > the >blue >color >is >the >area >that >
is >not >drawn >by >the >box. >
Making >your >own >Boxtypes>
Warning: >this >interface >may >change >in >future >
versions >of >fltk!>
You >can >define >your >own >boxtypes >by >making >a >
small >function >that >draws > the >box >and >adding >
it >to >the >table >of >boxtypes. >
The >Drawing >Function>
The >drawing >function >is >passed >the >bounding >box >
and >background >color > for >the >widget: >
>void xyz_draw(int x, int y, int w, int h, Fl_Color c) {
>...
>}
>
A >simple >drawing >function >might >fill >a >rectangle >
with >the >given >color > and >then >draw >a >black >
outline: >
>void xyz_draw(int x, int y, int w, int h, Fl_Color c) {
> fl_color(c);
> fl_rectf(x, y, w, h);
> fl_color(FL_BLACK);
> fl_rect(x, y, w, h);
>}
>
Adding >Your >Box >Type>
The >Fl::set_boxtype()> method >adds >or >replaces >
the >specified > box >type: >
>#define XYZ_BOX FL_FREE_BOXTYPE
>
>Fl::set_boxtype(XYZ_BOX, xyz_draw, 1, 1, 2, 2);
>
The >last >4 >arguments >to >Fl::set_boxtype()>
are >the >offsets >for > the >bounding >box >that >
should >be >subtracted >when >drawing >the >label >
inside >the >box. >
The >label()>, >align()>, >labelfont()>
, > labelsize()>, >and >labeltype()>
methods >control >the >labeling > of >widgets. >
label()>
The >label()> method >sets >the >string >that >
is >displayed >for >the > label. >For >the >
FL_SYMBOL_LABEL> and >image >label >types >the >
string >contains >the >actual >symbol >or >image >data. >
align()>
The >align()> method >positions >the >label. >
The >following > constants >are >defined >(they >may >be >
OR'd >together >as >needed): >
- FL_ALIGN_CENTER> - >center >the >label >in >
the >widget. >
- FL_ALIGN_TOP> - >align >the >label >at >the >
top >of >the >widget. >
- FL_ALIGN_BOTTOM> - >align >the >label >at >the >
bottom >of >the > widget. >
- FL_ALIGN_LEFT> - >align >the >label >to >the >
left >of >the >widget. >
- FL_ALIGN_RIGHT> - >align >the >label >to >the >
right >of >the > widget. >
- FL_ALIGN_INSIDE> - >align >the >label >inside >
the >widget. >
- FL_ALIGN_CLIP> - >clip >the >label >to >the >
widget's >bounding > box. >
- FL_ALIGN_WRAP> - >wrap >the >label >text >as >
needed. >
labeltype()>
The >labeltype()> method >sets >the >type >of >
the >label. > The > following >standard >label >types >
are >included: >
- FL_NORMAL_LABEL> - >draws >the >text. >
- FL_NO_LABEL> - >does >nothing >
- FL_SYMBOL_LABEL> - >draws >"@xyz" >labels, >see >
"> Symbol >Labels>" >
- FL_SHADOW_LABEL> - >draws >a >drop >shadow >
under >the >text >
- FL_ENGRAVED_LABEL> - >draws >edges >as >though >
the >text >is > engraved >
- FL_EMBOSSED_LABEL> - >draws >edges >as >thought >
the >text >is > raised >
To >make >bitmaps >or >pixmaps >you >use >a >method >
on >the > Fl_Bitmap>
or >Fl_Pixmap>
objects. >
Making >Your >Own >Label >Types>
Warning: >this >interface >is >changing >in >FLTK >
2.0!>
Label >types >are >actually >indexes >into >a >table >
of >functions >that >draw > them. > The >primary >purpose >
of >this >is >to >let >you >reuse >the >label()>
pointer >as >a >pointer >to >arbitrary >data >such >as >
a >bitmap >or >pixmap. >You > can >also >use >this >
to >draw >the >labels >in >ways >inaccessible >through >
the > fl_font> mechanisim >(e.g. >FL_ENGRAVED_LABEL>
) >or >with > program-generated >letters >or >
symbology. >
Label >Type >Functions>
To >setup >your >own >label >type >you >will >need >
to >write >two >functions >to > draw >and >measure >the >
label. > The >draw >function >is >called >with >a >
pointer > to >a >Fl_Label>
structure >containing >the > label >information, >the >
bounding >box >for >the >label, >and >the >label >
alignment: >
>void xyz_draw(Fl_Label *label, int x, int y, int w, int h, Fl_Align align) {
>...
>}
>
The >label >should >be >drawn >inside> this >
bounding >box, >even >if > FL_ALIGN_INSIDE> is >
not >enabled. > The >function >is >not >called >if >the >
label >value >is >NULL>. >
The >measure >function >is >called >with >a >pointer >
to >a > Fl_Label> structure >
and >references >to >the >width >and >height: >
>void xyz_measure(Fl_Label *label, int &w, int &h) {
>...
>}
>
It >should >measure >the >size >of >the >label >and >
set >w> and >h> to >the >size >it >
will >occupy. >
Adding >Your >Label >Type>
The >Fl::set_labeltype> method >creates >a >label >
type >using >your > draw >and >measure >functions: >
>#define XYZ_LABEL FL_FREE_LABELTYPE
>
>Fl::set_labeltype(XYZ_LABEL, xyz_draw, xyz_measure);
>
The >label >type >number >n> can >be >any >
integer >value >starting >at > the >constant >
FL_FREE_LABELTYPE>. > Once >you >have >added >the >
label > type >you >can >use >the >labeltype()>
method >to >select >your >label > type. >
The >Fl::set_labeltype> method >can >also >be >
used >to >overload > an >existing >label >type >such >
as >FL_NORMAL_LABEL>. >
The >FL_SYMBOL_LABEL> label >type >uses >the >
label()> string >to >look >up >a >small >drawing >
procedure >in >a >hash >table. > For > historical >
reasons >the >string >always >starts >with >'@'; >if >
it >starts >with > something >else >(or >the >symbol >
is >not >found) >the >label >is >drawn > normally: >
The >@ >sign >may >be >followed >by >the >following >
optional >"formatting" > characters, >in >this >order: >
- '#' >forces >square >scaling, >rather >than >distortion >
to >the > widget's > shape. >
- +[1-9] >or >-[1-9] >tweaks >the >scaling >a >little >
bigger >or > smaller. >
- [1-9] >- >rotates >by >a >multiple >of >45 >
degrees. > '6' >does > nothing, > the >others >point >in >
the >direction >of >that >key >on >a > numeric >keypad. >
Callbacks >are >functions >that >are >called >when >the >
value >of >a >widget > changes. >A >callback >function >
is >sent >a >Fl_Widget> pointer >of > the >
widget >that >changed >and >optionally >a >pointer >to >
data >of >some >sort: >
>void xyz_callback(Fl_Widget *w, void *data) {
>...
>}
>
The >callback()> method >sets >the >callback >
function >for >a > widget. > You >can >optionally >pass >
a >pointer >to >some >data >needed >for >the >
callback: >
>int xyz_data;
>
>button->callback(xyz_callback, data);
>
Normally >callbacks >are >performed >only >when >the >
value >of >the >widget > changes. >You >can >change >
this >using >the >
when()> method: >
>button->when(FL_WHEN_NEVER);
>button->when(FL_WHEN_CHANGED);
>button->when(FL_WHEN_RELEASE);
>button->when(FL_WHEN_RELEASE_ALWAYS);
>button->when(FL_WHEN_ENTER_KEY);
>button->when(FL_WHEN_ENTER_KEY_ALWAYS);
>button->when(FL_WHEN_CHANGED | FL_WHEN_NOT_CHANGED);
>
Shortcuts >are >key >sequences >that >activate >widgets >
(usually >buttons >or > menu >items). > The >shortcut()>
method >sets >the >shortcut >for >a > widget: >
>button->shortcut(FL_Enter);
>button->shortcut(FL_SHIFT + 'b');
>button->shortcut(FL_CTRL + 'b');
>button->shortcut(FL_ALT + 'b');
>button->shortcut(FL_CTRL + FL_ALT + 'b');
>button->shortcut(0); // no shortcut
>
The >shortcut >value >is >the >key >event >value >(the >
ASCII >value >or >one >of >the >special >keys >like >FL_Enter>) >
combined >with >any >modifiers >(like >shift, >alt, >and >
control). >
Contents
Previous
Next